This time, I've created a really simple text based game that allow the player to progress state to another state by choosing some options. Some options might take the player to the final state of the story and that's where the game end.
A. What I learnt from this course session are:
1. Importing image assets
To import an image asset, we just need to drag and drop the image from your directory explorer to the asset panel. And if you're working on a 2D game make sure the texture type is
"Sprite (2D and UI)" by selecting the image asset in the asset panel and checking the texture type in the inspector.
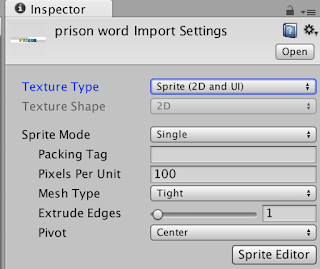 |
Unity Game Development - Checking Texture Type in Inspector Panel |
2. Adding GameObject to the scene
In this game, I'm using game object called UI Text. UI Text is an object to display text in the game. To add UI Text: Open Game Object menu -> UI -> Text
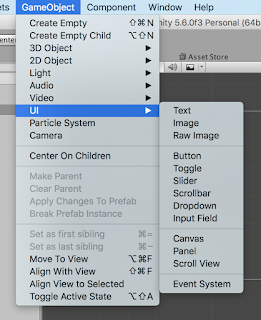 |
Unity Game Development - Adding UI Text to Game Scene |
3. Toggle layers
In Unity's Game Scene, there are several layers that you can toggle to be visible/invisible. You can find that toggle in the top right corner of Unity.
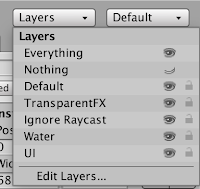 |
Unity Game Development - Toggle Layers |
4. Canvas
Canvas is where all the game will be operating in. So if you put GameObject such as Text UI outside canvas, that object won't be visible during game play mode.
5. Interacting with GameObjects in Scene Panel
In the upper-left of unity you can find some useful tools to interact with game object on the scene. Those tools are Hand (Shortcut Q), Move (Shortcut W), Rotate (Shortcut E), Scale (Shortcut R), and UI tool (shortcut T).
 |
Unity Game Development - Tools to Interact with Game Objects |
6. Setting camera background color
The camera uses blue as its default background color. We can change it easily just by selecting the Main Camera object in the Hierarchy and change the background color from the inspector panel. In this case, I changed the background color to be black.
7. Add a new component/script to UI Text object
If you want to give your UI text a behavior you can add a script to your game object. One way to add a script is:
1. Select your game object from Hierarchy (In case of my game, it is a UI Text object).
2. Go to your inspector panel and scroll to the bottom. You will find add component button on the bottom.
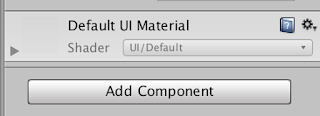 |
Unity Game Development - Add Component/Script to Game Object |
3. If you want to use existing script, choose "Scipts". But if you want to create new script, choose "New Script".
8. Properties in a assets class will be accessible trough inspector
In order to access your UI Text in your code, you need to create a property in your class for example:
Then to connect your property to the Game Object, you need to select your UI Text object from Hierarchy and then check your inspector (but before that you need to add script to your game object). From the inspector, you'll find a Text Controller (Script) component, and you can set the Text property to the UI Text object as seen in screen capture below.
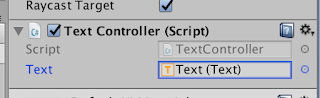 |
Unity Game Development - Connecting Text Property to UI Text Game Object |
9. There are two main views in Unity (Scene View and Game View)
As you build your game, you can switch from the Scene View to the Game View to preview how it will look in its platform-specific final build. Learn how to preview and test your game as you work. That makes it really fast and easy to try things out.
What are scenes and Game Objects?
You can think of a scene as a level of your game (but it could also be another element, like a main menu, for example). Game Objects are the environment, cameras, lights, and all the other elements in the scene.
Tips:
- To get into the center of game object: go to Hierarchy -> double clicks on the game object and you will see your game object in the scene view
- We can connect game object to the script by creating a public property in the script and then connect that property with GameObject by simply choosing from the inspector.
B. Developing Text 101
In the game development process itself, we are using what we call the Game Design Document (GDD). GDD is a document that outlines our game and its screens. We also learn about
Finite-state machine (FSM). FSM is an abstract machine that can be in exactly one of a finite number of states at any given time. It can change from one state to another in response to external inputs.
Game State Diagram
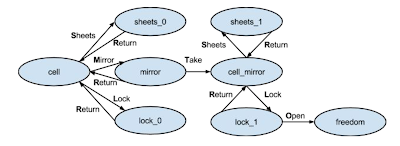 |
Unity Game Development - State Diagram 1 |
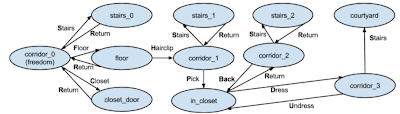 |
Unity Game Development - State Diagram 2 |
In order to transition between states, player need to press a key represent by the state. For example in the first state diagram, player starts in a "cell" state. If the player press "M" key, the player will go to "mirror" state. In code, each state is represented by enum as shown in snippet of code below.
In
Update() method, we handle all currentState of the player and create methods to handle the state transition. You might get an idea by looking at this snippet of code.
These are some screenshots of my game. In first screenshot, you can see when the player in the "cell" state and then transitioning to the second state Mirror by pressing M.
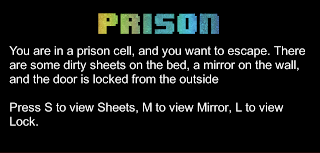 |
Unity Game Development - Player in First "cell" State |
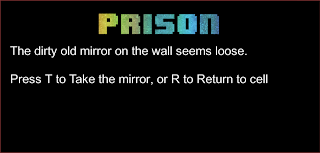 |
Unity Game Development - Player in Second "mirror" State |
If you are interested of making this kind of game please take a look at my Github repo below for a complete code:
https://github.com/ibanezang/text101
You can just clone the code and run it on your local machine. I hope you enjoy reading my writing. Can't wait to share more about
my game development journey! Thank you.